In this subject we will look at how the Angular Change Detection system works.
First of all, an Angular application is a tree of components, starting from App Component and the list of child components that you build all the way down.
If you look visually, your application will appear as a tree of components, starting from app-component as a root.
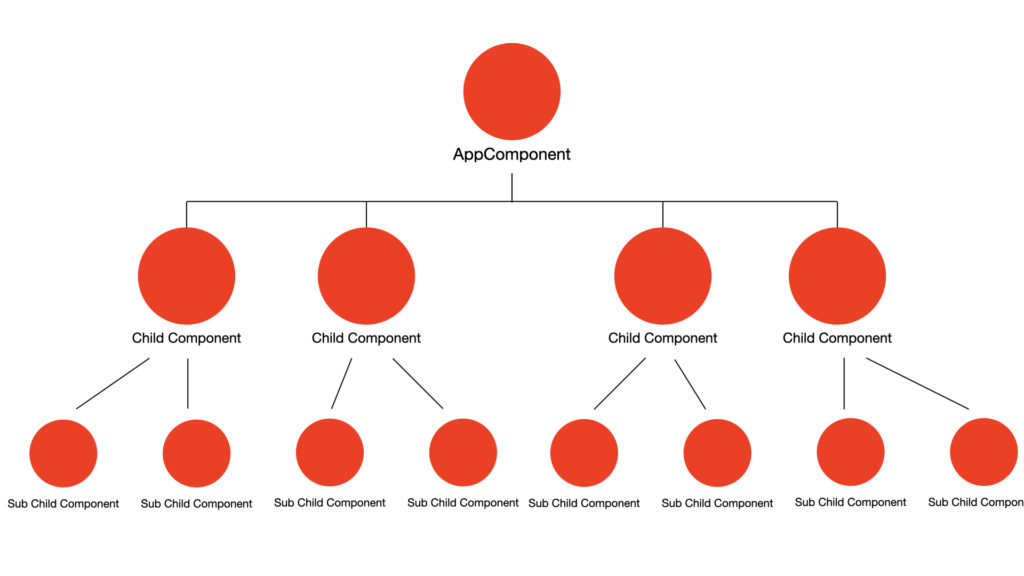
Behind the scenes, angular uses a low-level layer called View, which holds a reference to your associated component class.
Your component class basically encapsulates these views, so anything on these views like DOM updates are updated.
And each view has a state, which plays a major role in deciding whether to execute a change detection for that view and all of it’s child components or not.
One thing to understand is, Angular is a reactive system and change detection is at the heart of it’s core.
How does Angular compiler detect changes?
Angular compiler runs change detection with the view, it goes over all the bindings which in our case input properties. Next it begins evaluating the expressions like comparing it with previous values.
This is dirty checking, finally after the comparison is done, if changes are detected, the DOM will be updated with new values.
That’s the basic mechanism of Change Detection in Angular.
Application states can be triggered by three things;
- Timers – setTimeout, setInterval
- XHR – Fetching data from an API
- Events – Such as click, submit
Please watch this video for use case scenario.
Change Detection Strategy
Angular provides developer to alter the change detection strategy by providing a strategy, such as OnPush and Default.
If we don’t provide or set any strategy, Angular assumes the strategy as “Default”
@Component({ selector: 'app-drug', templateUrl: './drug.component.html', styleUrls: ['./drug.component.scss'], changeDetection: ChangeDetectionStrategy.OnPush, })
The OnPush Strategy
If your component is set with OnPush strategy, and the object that are bound through input property mutates. The change detection will not work for this component and all of it’s child component.
This is because, the OnPush compared with object references instead of value, since when object mutates, it’s reference doesn’t change, hence there is not change detection happened.
A mutable object with same reference will not work in ChangeDetectionStrategy.OnPush.
We can disable change detection by simply calling the detach() method and enable them by calling another method called detectChanges()
Now, in conclusion, Angular change detection is similar to angular js like comparing template expressions. But there is a difference, Change detection doesn’t not work as a continuous loop. Whereas in angularjs we call it as digest cycle, which is basically a loop.
The change detection mechanism is customizable in Angular using Change Detection Strategy as default or onPush.
I know I may not have covered all aspects of Change Detection, but if you feel you need more, please comment on this video.
In most cases, as a developer we should not worry about the “default” change detection strategy.
But we must keep in mind that if your app is going to handle plenty of data objects and it’s dependencies across components.
Especially in a case where a table consists of 100’s of records with pagination.
Thank You!